Part 1:
You are a programming intern at the student transfer counselor's office. Having heard you are taking CS 55, your boss has come to ask for your help with a task.
Students often come to ask her whether their GPA is good enough to transfer to some college to study some major and she has to look up the GPA requirements for a school and its majors in a spreadsheet to answer their question. She would like you to write a program that students can use themselves to get their answer. She is providing you with the spreadsheet data.
CS
Econ
English
UCLA
3.7
3.5
3.2
UCB
3.8
3.4
3.3
UCI
3.6
3.7
3.4
UCSD
3.5
3.3
3.0
You will write a program, TransferAdvisor.java, which asks the user for the school name, the major, and their GPA, and informs them whether their GPA is adequate for the school and major selected, or by how much they need to improve it. If a school or major is not in the spreadsheet, the user is informed. The school names and majors can be entered both as shown in the spreadsheet or in all lowercase letters. The “You need to improve your GPA by ### …” message should have 2 decimals precision for the GPA regardless of the student GPA entered.
Below are a few runs of the program:
Enter the school name: UCLA Enter the major: CS
Enter your GPA: 3.457
You need to improve your GPA by 0.24 to transfer to UCLA to study CS
—————–
Enter the school name: ucsd Enter the major: english Enter your GPA: 3.12
You could transfer to ucsd to study english
——————
Enter the school name: harvard Enter the major: CS
Enter your GPA: 3.1
harvard is not in the school system
——————
Enter the school name: ucla Enter the major: astrology Enter your GPA: 2.6
There is no astrology major at ucla
Hints:
– To avoid a lot of duplication with the code dealing with the GPA comparison and print outs, write a method that takes in the school name, the major name, the required GPA for that major in that school and the student GPA, and prints out either the “You could transfer …” or the “You need to
improve your GPA by …”.
– Avoid if/else if/… to match the school and the major. Switch/case makes the code cleaner.
Part 2:
Your boss has come to ask for your help with another task. She would like you to write a
simple program that encodes and decodes “secret messages” which she plans to demonstrate to elementary school students to kindle their interest in Computer Science.
The encoding/decoding scheme is very simple. Each letter is substituted by some other letter according to a given mapping as shown below.
// String letters = "abcdefghijklmnopqrstuvwxyz"; String enc = "kngcadsxbvfhjtiumylzqropwe";
For projectple, every 'a' becomes a 'k' when encoding a text, and every 'k' becomes an 'a' when decoding.
You will write a program, SecretMessage.java, which asks the user whether they would like to encode or decode a message, and then encodes or decodes the message using the mapping above.
Capital letters are mapped the same way as the lower case letters above, but remain capitalized.
For projectple, every 'A' becomes 'K' when encoding a text, and every 'K' becomes an 'A' when decoding. Numbers and other characters are not encoded and remain the same. The program repeatedly asks the user for input until the user quits.
Below is one run of the program:
Enter 1 to encode, 2 to decode, 3 to quit: 1 Enter the text to encode:
Santa Monica College Spring 2014 Lktzk Jitbgk Gihhasa Luybts 2014
Enter 1 to encode, 2 to decode, 3 to quit: 1 Enter the text to encode:
programming is fun uyisykjjbts bl dqt
Enter 1 to encode, 2 to decode, 3 to quit: 2 Enter the text to decode:
Lktzk Jitbgk Gihhasa Luybts 2014 Santa Monica College Spring 2014
Enter 1 to encode, 2 to decode, 3 to quit: 3 Good bye
Hints:
Think about decomposing the problem into simpler problems. You will likely find it helpful to
write a few very simple methods first:
– isLowerCaseLetter takes a char and returns true if that letter is a lower case letter (between 'a' and 'z'). isUpperCaseLetter and isLetter should work similarly.
– toLowerCase takes an upper case letter and returns the same letter in lower case. Do not use conditionals to implement this (if/else or switch). Instead, remember that a char is “a number” code. Print that number code for 'A', 'B', 'a', 'b' to see how it looks and come up with a very simple way to change an upper case letter to lower case. toUpperCase should work similarly.
– codeChar takes a char and a boolean flag. If the flag is true, it returns the encoded char according to the given mapping. If the flag is false, it returns the decoded char according to the given mapping. Do not use conditionals to do the mapping. Instead, notice that the letter 'a' is encoded to the first character of the given encoding map. The letter 'b' to the second,
and so on. For the decoding, you can write a decoding string by hand that you can use to map the encoded letters 'a' to 'z' to their decoded counterparts.
Given a String in Java you can use the charAt method to get the letter at a given position. For projectple:
String s = "projectple";
char first = s.charAt(0); // first is assigned 'e'
char second = s.charAt(1); // second is assigned 'x'
Notice that to get the character at the first position we pass the argument 0 to the charAt method and not 1.
– codeMessage takes a String and a boolean flag. If the flag is true, it prints the given message encoded. If the flag is false, it prints the given message decoded. This method just calls the codeChar method for every character in the String and prints the character that codeChar returns. It is really short and simple.
Given a String in Java you can use the length method to get the number of characters in that String. For projectple:
String s = "hello";
int len = s.length(); // len is assigned 5
Deliverables:
You should submit a zip file named project1_first_last.zip (where first and last are your first and last name) containing ONLY the 2 files below.
TransferAdvisor.java SecretMessage.java
How you get points:
– TransferAdvisor.java 40 points
– SecretMessage.java 60 points
How you lose points:
– If your code does not follow Java coding standards regarding naming of classes, variables, methods, constants, etc.
– If your code is not formatted properly and I find it hard to read.
– If your code is not commented properly:
– Every class file should have a header comment that includes your name and assignment number and briefly documents what the program does.
– If there are known deficiencies with your program such as known problems or incomplete features, these should be clearly listed in the header comment.
– Every method should have a method header comment that documents what the method does, what its parameters are, and what it returns.
– Every complex block of code should have at least a line comment documenting that code's intent.
– If your source code has print out statements you used for debugging or commented out code you used to try out ideas. Clean up your code before you submit. Do not leave clutter behind.
CS案例:java案例 case write a program代码案例北美案例
2018-03-01
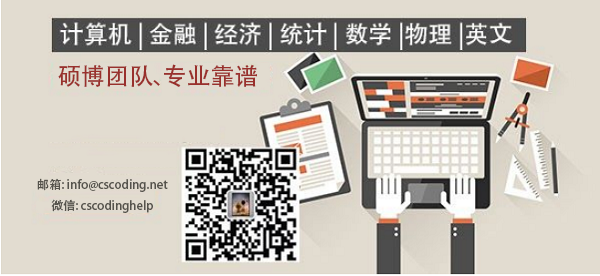