1 Introduction
Thisprojectwilltakesignificanttimetoimplement.Youcannotfinishitinasingle nightorweekend!Youwillneedtoplanyourtimecarefullyandworkoneachbitasthe topic iscovered.
Your task is to create a music media library. The library will be able to perform the following:
Store details of music stored on Records, Cassettes, and Digitally;
Read the library details from an input file;
Add songs to the library based on user input;
Calculate the total play time of all songs stored in the library;
Findaspecificsong(basedonfirstoccurrencenamematches)andprinttheplay instructions for thatsong.
Allowtheusertoselectamediatypeandoption,andthenprintnumberofsongs and total run time for thattype:
– Records, and the record playspeed.
– Digital, and the filetype.
– Cassette, and the tapeside.
? Save the library to a file.
2 TaskDetails
The final functionality of the music library is as follows:
2.1 DataValidation:
The details of all music must conform to the following specifications.
? All songs have the following pieces of information:
Name–mustnotbenulloranemptystring.
Artist – must not be null or an emptystring.
Duration–mustbearealnumberbetween0.0and9.59(inclusive).Thismustbe a valid .time. ie: 9.87 isinvalid.
? Records have the following additional information:
Track Number – Must be an integer between 0 and 20 (inclusive).
Play Speed – Must be one of the following strings, casing does not matter:
– “33 1/3 RPM”
– “45RPM”
– “78RPM”
? Digital tracks have the following additional information:
Type – Must be one of the following strings, casing does not matter:
– “wav”
–“mp3”
– “acc”
? Cassettes have the following additional information:
Track Number – Must be an integer between 0 and 20 (inclusive).
StartTime–Mustbearealnumberbetween0.0and160.0(inclusive).Thismust be a valid .time. ie: 5.89 isinvalid.
Side – Must be a character, A, or B, lowercase, or uppercase.
2.2 AddMusic:
Addsongstothelibrarybasedonuserinput,thesamevalidationastheprevioussection applies.
2.3 TotalDuration:
Calculate the total play time of all songs stored in the library;
2.4 PlayInstructions
Zero to Hero is track number 3 and the record is be played at 33 1/3 rpm. MakeaManOutofYourequiresthewavcodectoplay.
Be Out Guest is track number 2, it starts at 2.30 minutes, on side A.
The user should be able to see the play instructions for each song. An example for each of the 3 music types is shows below.
2.5 Search:
Findaspecificsong(basedonfirstoccurrencenamematches)andprinttheplayinstruc- tionsforthatsong.Thisisasimplelinearsearch,loopthrougheacharray,andprintthe instructions for the first (only the first) match. Matches should ignorecase.
2.5.1Search &Duration:
Allowtheusertoselectamediatypeandoption,andthenprintthenumberofsongsand total run time for thattype:
? Records, and the record play speed.
? Digital, and the file type.
? Cassette, and the tape side.
2.6 Input &Output
Input and output should be done using the techniques from the lectures and practical sessions.
2.6.1 InputFile
Youwillberequiredtoloadthemusiclibraryfromacsvfile(thenameofwhichissupplied by the user). For each music type the csv file will conform to the followingformat:
Record – R,,,,,
Digital – D,,,,
Cassette – C,,,,,,
R,Zero to Hero,The Muses,4.37,3,33 1/3 RPM D,Make a Man Out of You,Mulan and Chang,3.57,WAV C,Be Our Guest,The Dishes,2.57,2,2.3,a
ThefirstletterofeachrowmustbeR,D,orC(inanycase),thisletterindicatesthetype of music the row is for. Below is an exampleinput:
Anymalformedrowsshouldbediscardedwhenreadingthefileandanappropriatemessage displayedtotheuserinformingthemofsuch,theremainderofthefileshouldcontinueto beread.Thefileitselfmayalsobeempty,youralgorithmshouldcheckforthisandprint an appropriate errormessage.
2.6.2 OutputFile
Your program must be able to save the current music library, to a file that conforms to the same format as the input file. That is, the generated output file must be able to be used on the next run of the program to “restore” the library to the same state. All real numbers should be formatted to 2 decimal places.
2.6.3 UserInput
All user input must be validated where appropriate.
2.7 UserOutput
All output to the user should be formatted in a pleasant, easy to read manner. This include prompts for input. All real numbers should be formatted to 2 decimal places.
2.8 ErrorHandling
Under no circumstance should the user be aware of exception handling, this includes having unhandled exceptions, and the user seeing any form of a stack trace.
All error messages displayed to the user should be meaningful to a non-programmer.
3 Documentation
You must thoroughly document your code using block comments (/*…*/) andin-line comments (//…). For each Class, you must place a comment at the top of the file containingyourname,thefilename,thepurposeoftheclassandanymodificationhistory. For eachmethod you write you mustplaceacommentimmediatelyaboveitexplaining its purpose, its import and export values, and how it works. Other comments must be included asnecessary.
4 SubmissionRequirements
projects should be submitted online via Moodle. Your submission should include:
The Design:
Pseudocode implementation of the algorithms.
TheCode:
.javafilesimplementingyourpsuedocodedesign(Donotsubmit.classfiles.)
.java files used to test your program
TheDocumentation
A PDF report, as outlined Below
4.1Report
Yoursubmissionmustincludeaoneandahalf(1.5)pagepagereport.Inthereportyou must discuss thefollowing:
? Justify your menu implementation decisions.
? Justify your approach to datavalidation.
?Justifyyourdesigndecisionsinregardstowhatfunctionalitywasplacedinthe container classes, and what functionality was placedelsewhere.
? Discuss any complications inheritance introduced in your design.
? Discuss and justify any down casting present in our code.
? Discussanychallengesyouhadinyourimplementation/design.
? Discussanychallengesyouhadinyourimplementation/design.
Youareresponsibleforensuringthatyoursubmissioniscorrectandnotcorrupted. You may make multiple submissions, but only your latest submission will be marked. You shoulddownloadyoursubmission,extractthecontentsandensureitstillcompiles/runs.
Please note that code should adhere to standard Java coding style and practices
5 Marking
ThepseudocodealgorithmandJavaimplementationwillbothbemarked.Yourjavacode willbeassessedbyitssuitabilityasavalidimplementationofyoursuppliedpseudocode. Thedemonstrationrequirementsforeachsubmissionaredescribedabove.Markswillbe allocatedfortheappropriatechoicesofclasses,theoverallfunctionality,andadheringto best programming practices as discussed in the lectures andpracticals.
Quizzes:
You cannotpasstheprojectwithoutattendingyourweeklytutorialandattempting yourquizzes.Thisprojectwillbeweightedby
计算机代码案例java案例 Object Oriented Program De
2018-09-18
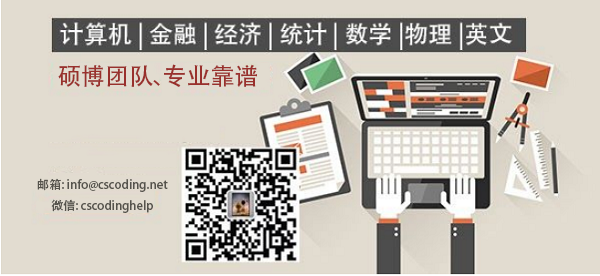