A Dolphin Population Model
Build a model for a dolphin population over 150 years. Model Assumptions and Input:
• Dolphins live for an average of 35 years, with a standard deviation of σ = 5 years.
• Dolphins procreate starting at the age of 8 until death. They produce no more than 1 calf every 5 years (assuming gestation length is zero).
• No dolphins can procreate with another that share the same set of parents and must procreate with other dolphins that are within 10 years of their own age and of the opposite sex. Male and female calves are equally likely: P(male) = 1 – P(female) = 0.5.
• No new calves can be named the same as any other dolphin, dead or alive.
• Start with an initial population of 4 dolphins, two females and two males, all at the age of 0.
Part I: Preparatory Steps
1. Use a webservice to make two data files with a unique name in each line, one for the males and the other the females. A good place to start:
http://www.prokerala.com/kids/baby-names/boy/page-1.html and
http://www.prokerala.com/kids/baby-names/girl/page-1.html
Download lists of male and female names from the above source. Write a function that retrieves
~ 7,500 names for both the males and females and save them in two separate data files (usually, with the extension .dat). At the moment this website has ~100 names per page, which means you need to download 75 pages worth of names.
2. Use the name data files made in the above step, write a name generating function. You should use yield rather than return in this function so that each time it is called, it will return the next name. This function should take sex as an input parameter.
When you run out of unique names, you are allowed to add a middle name. The middle name can be a random string of ten letters. (No, we don’t care that much about middle names, not those for dolphins anyway!) You can do a quick calculation and convince yourself that, with middle names generated this way, the probability of having two dolphins with the same
name is extremely low.
3. Create a class Dolphins() that should include at least the following attributes:
- name
- sex
- age # initialized to 0 of course.
- mother
- father
- years_since_procreation
- death # That is, how long an individual dolphin lives # and it should follow a Gaussian distribution.
Besides init it should have two other methods:
-
age_record
which keeps track of the dolphin's age and years since procreation, and determines when the dolphin dies.
and
-
request_procreation
which determines whether this dolphin and another can procreate.
Part II: Model Output
Write a main program called dolphin_pop_model.py that
A) Finds the average number of living dolphins and the standard deviation for each of the 150 years. You need to evolve the dolphin population at least 10 times to find the mean and the standard deviation. While running the trials, you should print out the information for the population every 25 years, for each trial. At the one hundred year mark, also report the
number of living dolphins at the end of that year and the total number of births since the very beginning of the trial, like this:
Trial No. 7 ##################################################
entering year 0 with 4 dolphins, with 0 breeding. ##################################################
entering year 25 with 14 dolphins, with 5 breeding. ##################################################
entering year 50 with 39 dolphins, with 13 breeding. ##################################################
entering year 75 with 104 dolphins, with 35 breeding. ##################################################
entering year 100 with 316 dolphins, with 114 breeding. at year 100, there are 330 living dolphins.
there have been 430 births, in total. ##################################################
entering year 125 with 1052 dolphins, with 381 breeding. ##################################################
at year 149, there are 3225 living dolphins.
**************************************************
Trial No. 8 ##################################################
entering year 0 with 4 dolphins, with 0 breeding. ##################################################
entering year 25 with 13 dolphins, with 4 breeding. ##################################################
entering year 50 with 28 dolphins, with 15 breeding. ##################################################
entering year 75 with 84 dolphins, with 24 breeding. ##################################################
entering year 100 with 267 dolphins, with 93 breeding. at year 100, there are 280 living dolphins.
there have been 354 births, in total. ##################################################
entering year 125 with 904 dolphins, with 316 breeding. ##################################################
at year 149, there are 2924 living dolphins.
…
Note that each trial ends at the end of year 149, since we start at year 0.
Finally, plot the average population size vs. year with the “error snake” around the mean. (the red band around the curve in the plot below).
Suggestion: use matplotlib.pyplot.fill_between() — see documentation for matplotlib.pyplot. You need to label the axes and give the plot an appropriate title. Save it as population_growth.pdf.
Below is an example:
image.png
B) Finds the minimum probability of producing males (i.e., P(male)) such that the population does not die out in 150 years. One way to do this is to systematically increase P(male) from 0 to 0.5 by 0.01 each time until the number of living dolphins is greater than zero at the end of year 149. You need to evolve the dolphin population at least five times and report the average minimum probability for P(male).
C) Randomly picks a dolphin (“The Main Character”) around year 70, and builds and plots a genealogy tree that shows all dolphins at the current and parent generations that are directly related to the Main Character. More specifically:
The Main Character: in the middle level.
Parents of the Main Character: one level higher.
Full Siblings of the Main Character: arrayed on the same level as the Main Character.
Half Siblings of the Main Character: arrayed one level below. Like this:
image.png
You do not need to print the words “Parents”, “Full Siblings”, and “Half Siblings” on your genealogy tree plot — I have included them on this example to help you understand how it should be structured. Save it as genealogy.pdf.
For the original population of four dolphins, you can name their parents however you would like, as long as that does not prevent the four dolphins from procreating.
CS案例之Python模型 实现:A Dolphin Population Model
2019-01-15
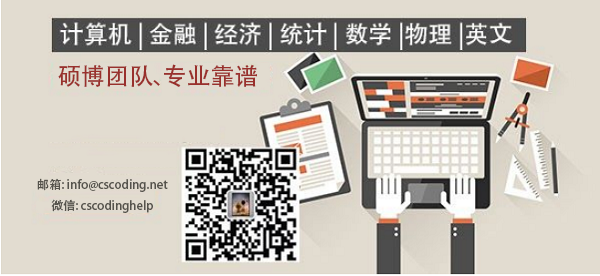